Radio Towers and Computers
The goal for this week was to finish creating the remain two objectives. Here is a short recap of the three objectives our player will need to manage:
- Keep Generator fueled
- Repair Radio Tower when damaged
- Reset Computer Equipment at the house
With the help of our redesigned objective system, creating the Radio Tower and Computer objectives was very straight forward. I created both objectives as child blueprints of our InteractiveObjectives_BP.
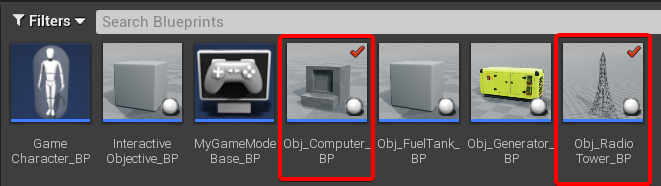
I also decided to create delegates for the Computer and Radio objectives similar to how I created the Power delegates earlier. Most of the following code does the same thing under different names. Essentially, we will be able to bind function calls to objective events. Similar to how we bind PoweredLights to the Generator objective. I cannot currently think of a situation we would need these for the Radio and Computer objectives, but I figured I might as well add them just in case.
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FPowerActivate);
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FPowerDeactivate);
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FRadioActivate);
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FRadioDeactivate);
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FComputerActivate);
DECLARE_DYNAMIC_MULTICAST_DELEGATE(FComputerDeactivate);
...
public:
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FPowerActivate PowerActivate;
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FPowerDeactivate PowerDeactivate;
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FRadioActivate RadioActivate;
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FRadioDeactivate RadioDeactivate;
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FComputerActivate ComputerActivate;
UPROPERTY(BlueprintAssignable, Category = "EventDispatchers")
FComputerDeactivate ComputerDeactivate;
// Function used for calling PowerActivate delegate
UFUNCTION(BlueprintCallable, Category = "Power Function")
void TurnPowerOn();
// Function used for calling PowerDeactivate delegate
UFUNCTION(BlueprintCallable, Category = "Power Function")
void TurnPowerOff();
UFUNCTION(BlueprintCallable, Category = "Power Function")
bool GetPowerState();
UFUNCTION(BlueprintCallable, Category = "Radio Function")
void TurnRadioOn();
UFUNCTION(BlueprintCallable, Category = "Radio Function")
void TurnRadioOff();
UFUNCTION(BlueprintCallable, Category = "Radio Function")
bool GetRadioState();
UFUNCTION(BlueprintCallable, Category = "Computer Function")
void TurnComputerOn();
UFUNCTION(BlueprintCallable, Category = "Computer Function")
void TurnComputerOff();
UFUNCTION(BlueprintCallable, Category = "Computer Function")
bool GetComputerState();
void AMyGameModeBase::TurnPowerOn() {
PowerActivate.Broadcast();
isPowerOn = true;
}
void AMyGameModeBase::TurnPowerOff() {
PowerDeactivate.Broadcast();
isPowerOn = false;
}
bool AMyGameModeBase::GetPowerState()
{
return isPowerOn;
}
void AMyGameModeBase::TurnRadioOn()
{
RadioActivate.Broadcast();
isRadioOn = true;
}
void AMyGameModeBase::TurnRadioOff()
{
RadioDeactivate.Broadcast();
isRadioOn = false;
}
bool AMyGameModeBase::GetRadioState()
{
return isRadioOn;
}
void AMyGameModeBase::TurnComputerOn()
{
ComputerActivate.Broadcast();
isComputerOn = true;
}
void AMyGameModeBase::TurnComputerOff()
{
ComputerDeactivate.Broadcast();
isComputerOn = false;
}
bool AMyGameModeBase::GetComputerState()
{
return isComputerOn;
}
Blueprint time
The blueprint code can be a little messy because of the timers. Put simply, if the Radio Tower or Computer are broken, the player has the option to repair them by holding the interact key (E). When repaired, a timer is set for when the objects will need to be repaired again. The object will also update visually to show its status.
The following blueprint code is identical. I considered making a proper parent for them, but considered it unnecessary for just two classes with such simple logic.
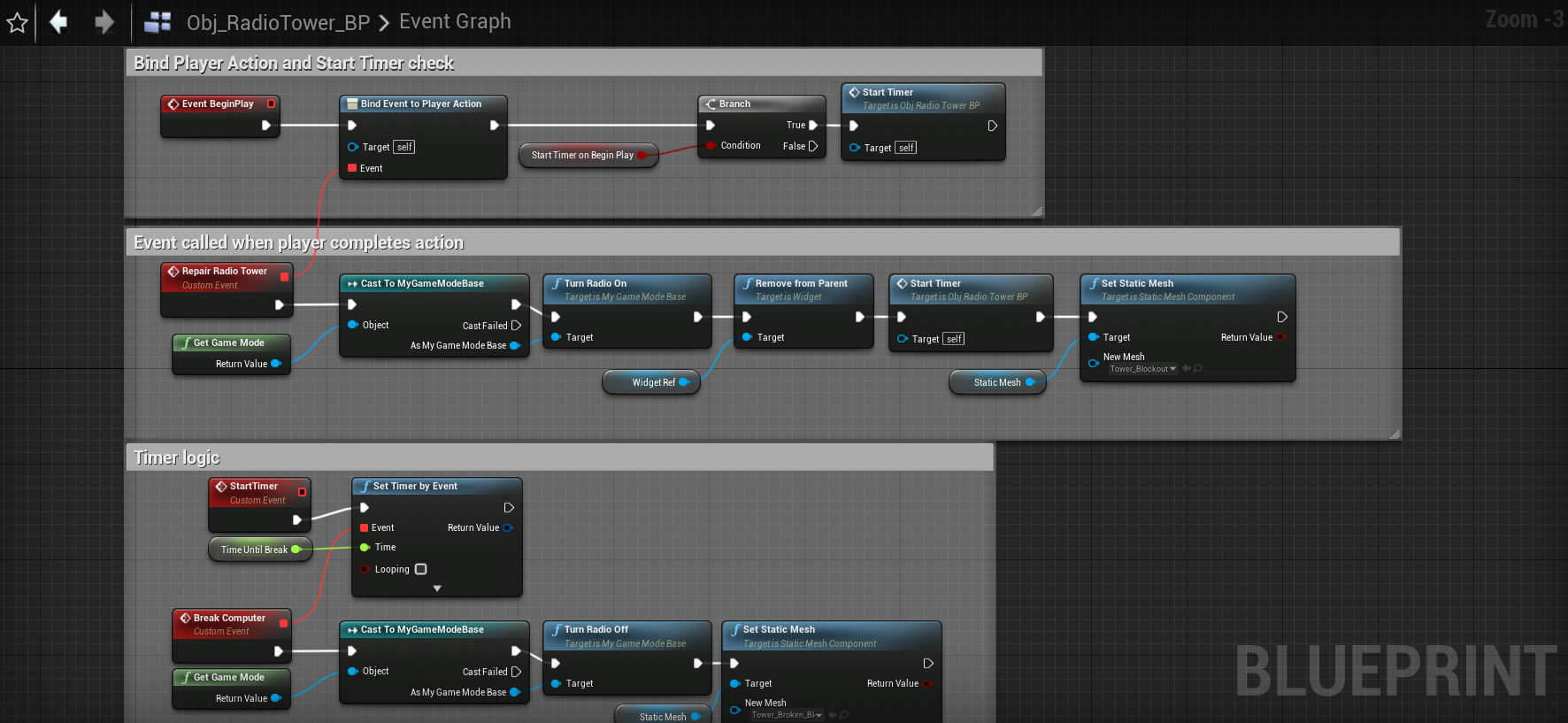
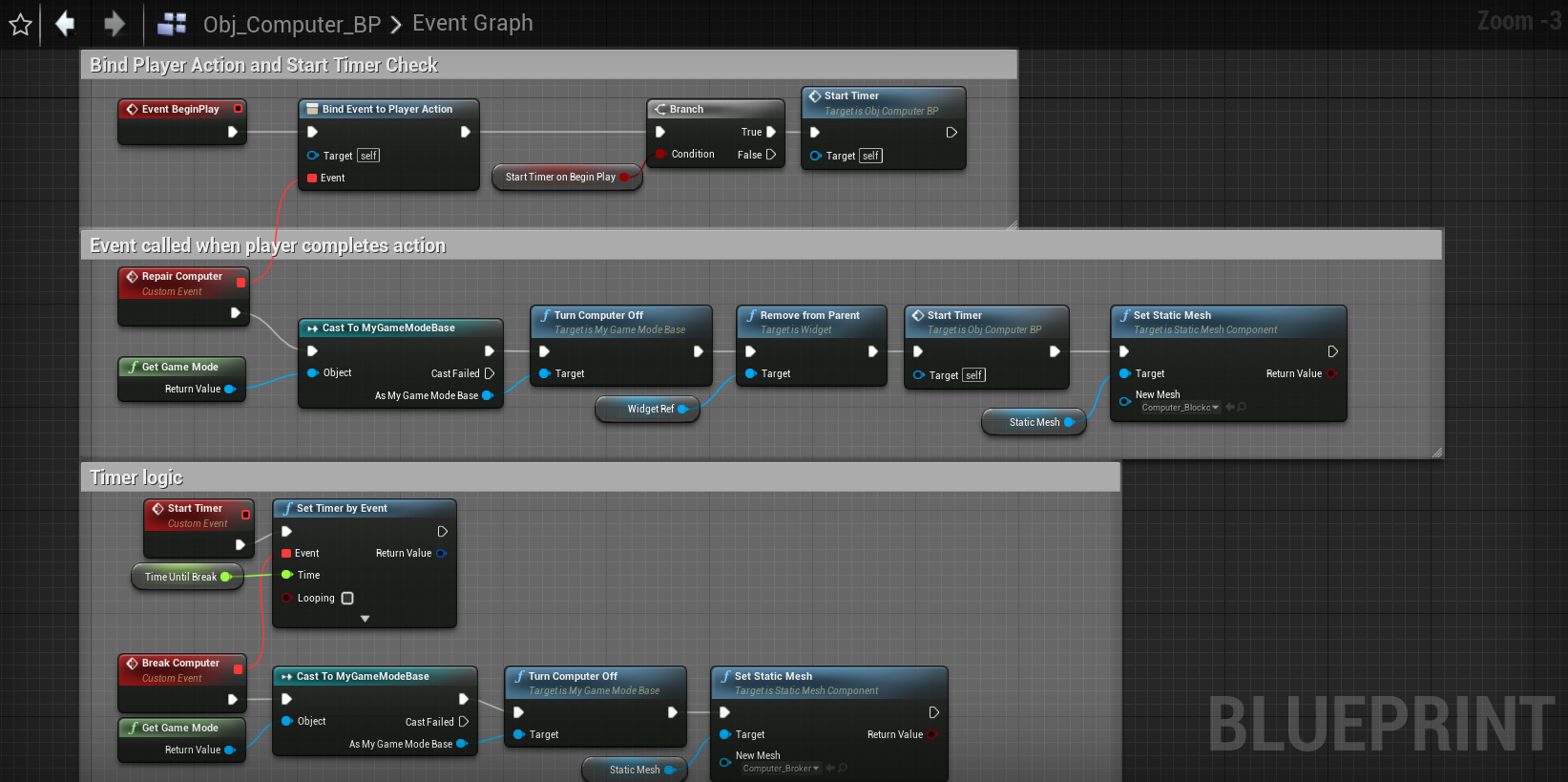
As you can see, basically Identical. Note that at the end of the “Repair Computer” and “Break Computer” Events we update our static mesh. This allows for better visual feedback for our player. We can also check a boolean value on instances of our blueprints to decide if we want the timers to start at the beginning of the game or be manually called. This will be helpful when designing the tutorial.
I also did an override on the ShouldShowWidget function from our parent. Again, the code is basically the same so I will just show the Computer implementation here

Demo time!

As you can see, it works! The Radio Tower and Computer delegates are also being updated in the background for future use.
With the objectives out the way, the goal for next week will be creating our scary AI enemy that will be watching and chasing the player. I was hoping to start working on the AI this week, but after watching some tutorials for Unreal Engine AI, I decided I will need much more time to work on the AI.
That is it for this week. Thanks for reading!